목표
띄어쓰기로 구분된 숫자 배열을 벡터에 각각 저장하고자 함.
방법 개요
1. 띄어쓰기로 구분된 숫자를 string으로 입력받기
2. 입력받은 string을 띄어쓰기를 구분자로 하여 쪼개기
3. 나눈 값들을 벡터에 저장하기
세부 방법
1. 띄어쓰기로 구분된 숫자를 string으로 입력받기
cin으로 입력받는 경우, 다음과 같이 처음으로 띄어쓰기가 나타나는 부분 이후로는 저장이 되지 않는다.
(cin의 특성이 그렇습니다.)
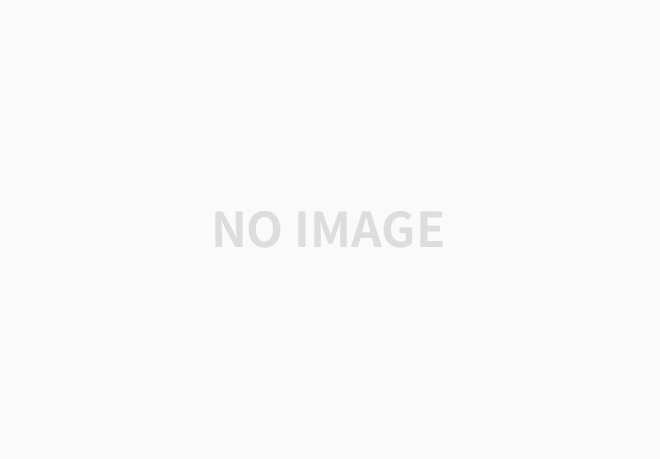
#include <iostream>
#include <string>
using namespace std;
int main()
{
std::string orgString;
cout << "'1 12 34'를 입력하세요." << endl;
cout << "\n";
cin >> orgString; // "1 12 34" 입력
cout << orgString << endl; // "1" 만 출력된다.
return 0;
}
이를 해결하기 위해서는 다음과 같이 std::getline을 이용해야 한다.
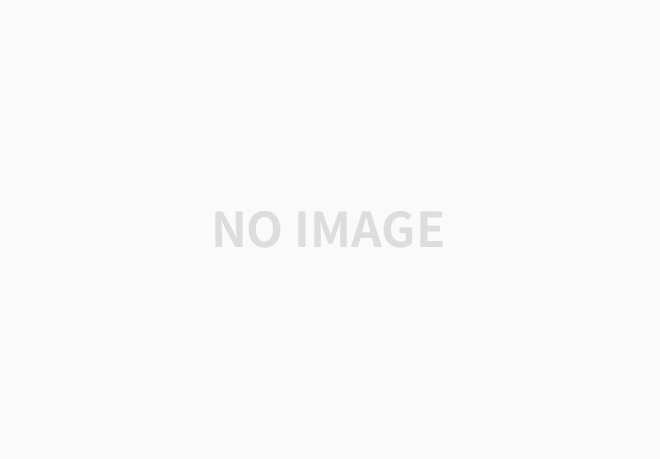
#include <iostream>
#include <string>
using namespace std;
int main()
{
std::string orgString;
cout << "'1 12 34'를 입력하세요." << endl;
cout << "\n";
std::getline(std::cin, orgString); // "1 12 34" 입력
cout << orgString << endl; // "1 12 34"가 출력된다.
return 0;
}
en.cppreference.com/w/cpp/string/basic_string/getline
std::getline - cppreference.com
template< class CharT, class Traits, class Allocator > std::basic_istream & getline( std::basic_istream & input, std::basic_string & str,
en.cppreference.com
2. 입력받은 string을 띄어쓰기를 구분자로 하여 쪼개기
string을 나누는 방법으로 <sstream>의 stringstream을 사용하였다.stringstream은 문자열에서 원하는 자료형의 데이터를 추출할 때 사용한다.
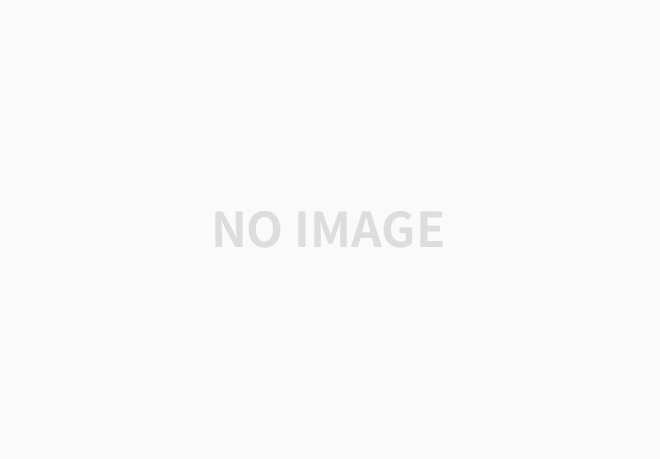
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
std::string orgString;
cout << "'1 12 34'를 입력하세요." << endl;
cout << "\n";
std::getline(std::cin, orgString); // "1 12 34" 입력
cout << orgString << endl; // "1 12 34"가 출력된다.
cout << "\n";
cout << "입력한 숫자는 ";
stringstream orgNum(orgString);
int a, b, c;
orgNum >> a >> b >> c;
cout << a << " " << b << " " << c << " 입니다." << endl;
return 0;
}
stringstream을 통해 받을 자료형을 지정해 줄 수 있는데, 위에서처럼 자료형이 모두 int가 아닌 다른 게 섞여 있으면 처음 int 부분만 제대로 저장된다. (다음과 같이 a, b, 55는 쓰레기 값이 나오게 된다. 즉 자료형을 정확히 지정해줘야 한다.)
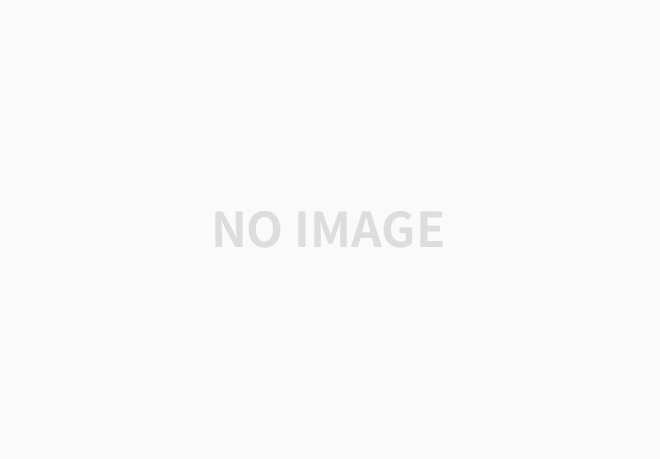
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
std::string orgString;
cout << "'1 12 34 a b 55'를 입력하세요." << endl;
cout << "\n";
std::getline(std::cin, orgString); // "1 12 34 a b 55" 입력
cout << orgString << endl; // "1 12 34 a b 55"가 출력된다.
cout << "\n";
cout << "입력한 숫자는 ";
stringstream orgNum(orgString);
int a, b, c, d, e, f;
orgNum >> a >> b >> c >> d >> e >> f;
cout << a << " " << b << " " << c << " " << d << " " << e << " " << f << " 입니다." << endl;
return 0;
}
a와 b의 자료형을 문자 자료형으로 바꾸면 다음과 같이 전부 다 잘 출력이 된다.
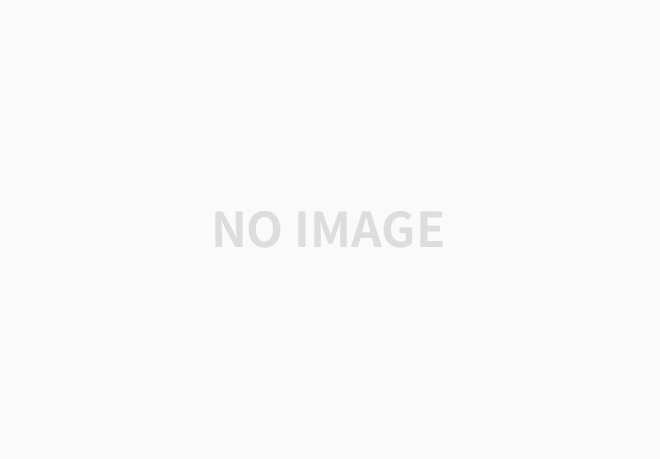
www.cplusplus.com/reference/sstream/stringstream/
stringstream - C++ Reference
class std::stringstream typedef basic_stringstream stringstream; Input/output string stream Stream class to operate on strings. Objects of this class use a string buffer that contains a sequence of characters. This sequence of characters can be a
www.cplusplus.com
3. 나눈 값들을 벡터에 저장하기
2번 프로세스에서 string을 띄어쓰기를 구분자로 하여 나눴고, 이제 이 값을 vector에 넣어보도록 하자. vector의 가장 큰 장점은 크기를 설정해주지 않아도 된다는 것이다. 따라서 vector를 사용하였다.
while (orgNum >> newNum) 을 사용하여 자료형이 int가 아닐 때까지 쪼개고, 이렇게 나눈 것을 벡터에 넣어 준다.
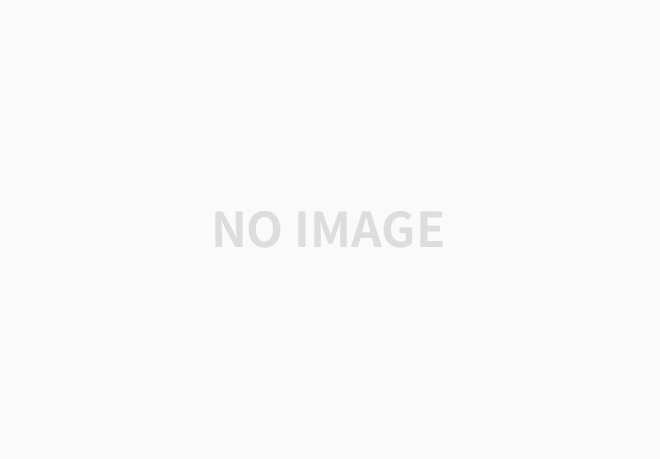
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
using namespace std;
int main()
{
std::string orgString;
cout << "'1 12 34 a b 55'를 입력하세요." << endl;
cout << "\n";
std::getline(std::cin, orgString); // "1 12 34 a b 55" 입력
cout << orgString << endl; // "1 12 34 a b 55"가 출력된다.
cout << "\n";
cout << "입력한 숫자는 ";
stringstream orgNum(orgString);
int newNum;
vector<int> numVec;
while (orgNum >> newNum)
{
numVec.push_back(newNum);
cout << newNum << " ";
}
cout << "입니다." << endl;
cout << "총 " << numVec.size() << "개의 숫자를 입력하셨습니다." << endl;
return 0;
}
참고
mannlim - Overview
mannlim has 6 repositories available. Follow their code on GitHub.
github.com
댓글